Mrrrr's Forum (VIEW ONLY)
Un forum care ofera solutii pentru unele probleme legate in general de PC. Pe langa solutii, aici puteti gasi si alte lucruri interesante // A forum that offers solutions to some PC related issues. Besides these, here you can find more interesting stuff.
|
Lista Forumurilor Pe Tematici
|
Mrrrr's Forum (VIEW ONLY) | Reguli | Inregistrare | Login
POZE MRRRR'S FORUM (VIEW ONLY)
Nu sunteti logat.
|
Nou pe simpatie: Sophya pe Simpatie
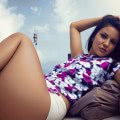 | Femeie 25 ani Bucuresti cauta Barbat 25 - 54 ani |
|
Mrrrr
AdMiN
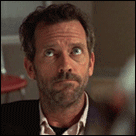 Inregistrat: acum 18 ani
Postari: 2257
|
|
I have 2000 folders, each containing 1 or more files and folders. Since I want to treat them separately, as in process the 1-file folders first, then the others separately, I need to move all folders containing just 1 file - not 2+ files, and not 1+ subfolders which may contain 1+ files themselves.
I didn't want to dive into moving the 1-file inside each folder, because this could lead to a rather "bulky" code and multiple errors could be involved - file naming conflicts, lots of remaining empty folders to deal with separately.
Source of all codes below: ChatGPT
The codes below will work for paths containing the "!" character. I have a tendency to use this in my folder paths, so I asked ChatGPT for a solution that works for such folders (the normal solution would not recognize the "!" character within the path).
Simply replace the 2 paths with your own, then paste the code into a Command Prompt window ran as Administrator:
Code 1 - Move folders containing 1 file
@echo off setlocal
REM Set the source and destination directories set "sourceDir=PATH_TO_SOURCE_DIRECTORY" set "destDir=PATH_TO_DESTINATION_DIRECTORY"
REM Navigate to the source directory cd /d "%sourceDir%"
for /d %%D in (*) do ( set "count=0" set "subfolder=0" for %%F in ("%%D\*") do ( if exist "%%F\*" ( set /a subfolder+=1 ) else ( set /a count+=1 ) ) call :checkAndMove "%%D" ) goto :eof
:checkAndMove set "folderName=%~1" if %count% equ 1 if %subfolder% equ 0 ( echo Moving "%folderName%" to "%destDir%" move "%folderName%" "%destDir%" if errorlevel 1 echo Failed to move "%folderName%" to "%destDir%" ) goto :eof
endlocal |
Code 2 - Rename files with folder names - OPTIONAL, OF COURSE
@echo off setlocal enabledelayedexpansion
REM Set the source directory set "sourceDir=PATH_TO_SOURCE_DIRECTORY"
REM Navigate to the source directory cd /d "%sourceDir%"
REM Process each subfolder for /d %%D in (*) do ( set "folderName=%%D" REM Rename files inside the folder to match the folder name for %%F in ("%%D\*") do ( if not "%%~nxF" == "%folderName%" ( set "fileExt=%%~xF" set "newFileName=%%D!fileExt!" echo Renaming "%%F" to "!newFileName!" ren "%%F" "!newFileName!" if errorlevel 1 echo Failed to rename "%%F" to "!newFileName!" ) ) )
endlocal |
_______________________________________

|
|
pus acum 9 luni |
|
Mrrrr
AdMiN
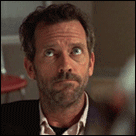 Inregistrat: acum 18 ani
Postari: 2257
|
|
Again, simply replace the 2 paths with your own, then paste the code into a PowerShell window ran as Administrator:
Code 1 - Move folders containing 1 file - PowerShell alternative
# Set the source and destination directories $sourceDir = "PATH_TO_SOURCE_DIRECTORY" $destDir = "PATH_TO_DESTINATION_DIRECTORY"
# Navigate to the source directory Set-Location -Path $sourceDir
# Get all subfolders $subfolders = Get-ChildItem -Directory
foreach ($folder in $subfolders) { $files = Get-ChildItem -Path $folder.FullName -File $subfoldersInside = Get-ChildItem -Path $folder.FullName -Directory
# Check if the folder contains exactly one file and no subfolders if ($files.Count -eq 1 -and $subfoldersInside.Count -eq 0) { $file = $files[0] $newFileName = "$($folder.Name)$($file.Extension)" $newFilePath = Join-Path -Path $folder.FullName -ChildPath $newFileName
# Rename the file to match the folder name - NOT ENABLED BY DEFAULT # Rename-Item -Path $file.FullName -NewName $newFileName -ErrorAction Stop
# Move the folder to the destination directory $destinationPath = Join-Path -Path $destDir -ChildPath $folder.Name Move-Item -Path $folder.FullName -Destination $destinationPath -ErrorAction Stop
Write-Output "Moved folder '$($folder.Name)' to '$destinationPath'" } } |
Code 2 - Rename files with folder names - PowerShell alternative
# Set the source directory $sourceDir = "PATH_TO_SOURCE_DIRECTORY"
# Navigate to the source directory Set-Location -Path $sourceDir
# Get all subfolders $subfolders = Get-ChildItem -Directory
foreach ($folder in $subfolders) { # Get files in the current folder $files = Get-ChildItem -Path $folder.FullName -File
foreach ($file in $files) { # Construct the new file name based on the folder name and the file extension $newFileName = "$($folder.Name)$($file.Extension)" $newFilePath = Join-Path -Path $folder.FullName -ChildPath $newFileName
# Check if the new file name is different from the current file name if ($file.FullName -ne $newFilePath) { # Rename the file to match the folder name Rename-Item -Path $file.FullName -NewName $newFileName -ErrorAction Stop
Write-Output "Renamed file '$($file.FullName)' to '$newFilePath'" } } } |
Code 3 - Rename files with folder names whilst keeping any year if it exists in the file names, before renaming
# Set the source directory $sourceDir = "PATH_TO_SOURCE_DIRECTORY"
# Navigate to the source directory Set-Location -Path $sourceDir
# Get all subfolders $subfolders = Get-ChildItem -Directory
foreach ($folder in $subfolders) { # Get files in the current folder $files = Get-ChildItem -Path $folder.FullName -File
foreach ($file in $files) { # Extract the year from the file name using a regular expression if ($file.Name -match '(\d{4})') { $year = $matches[1] } else { $year = '' }
# Construct the new file name based on the folder name and the file extension if ($year) { $newFileName = "$($folder.Name) ($year)$($file.Extension)" } else { $newFileName = "$($folder.Name)$($file.Extension)" } $newFilePath = Join-Path -Path $folder.FullName -ChildPath $newFileName
# Check if the new file name is different from the current file name if ($file.FullName -ne $newFilePath) { # Rename the file to match the folder name and year Rename-Item -Path $file.FullName -NewName $newFileName -ErrorAction Stop
Write-Output "Renamed file '$($file.FullName)' to '$newFilePath'" } } } |
_______________________________________

|
|
pus acum 9 luni |
|
Mrrrr
AdMiN
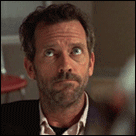 Inregistrat: acum 18 ani
Postari: 2257
|
|
To clear the PowerShell or Command Prompt window, simply type cls and press enter.
_______________________________________

|
|
pus acum 9 luni |
|